Understanding and Fixing the “You will need to pass in an i18next instance by using initReactI18next” Error

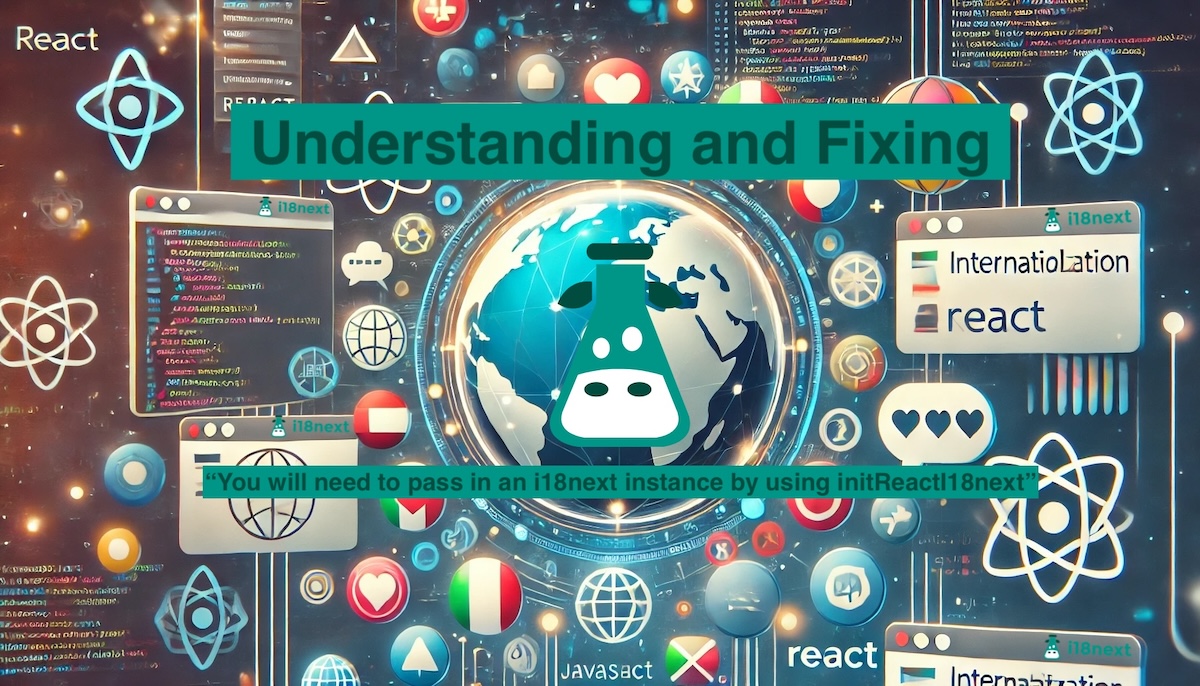
When working with internationalization in React using i18next
and its React bindings (react-i18next
), you might encounter the following error message:
react-i18next:: You will need to pass in an i18next instance by using initReactI18next
At first glance, this error may seem puzzling. It implies that the translation hook or component isn’t receiving the necessary i18next
instance. In this post, we’ll explore several common scenarios that trigger this error and discuss how to resolve them.
What Does the Error Mean?
In essence, react-i18next
needs to have an i18next
instance that has been “enhanced” with the React binding (via initReactI18next
). If this isn’t set up correctly, hooks like useTranslation
or components like <Trans>
won’t work because they can’t access the proper context. This is why the error instructs you to “pass in an i18next instance.”
You can even see in the source code of react-i18next
’s useTranslation
hook that if no i18next instance is found (i.e. if it isn’t provided via context or props), it warns:
“useTranslation: You will need to pass in an i18next instance by using initReactI18next”
– react-i18next/src/useTranslation.js at L41
Common Scenarios and How to Fix Them
1. Missing or Improper i18next Initialization
Problem:
If you forget to import and initialize your i18n configuration at the root of your app — or you neglect to wrap your application in an <I18nextProvider>
— the i18next instance will not be available to your translation hooks.
Solution:
Make sure your i18n file calls:
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
i18n.use(initReactI18next).init({
// ...your options here
});
export default i18n;
Then import this file in your top‑level entry point (e.g. in your index.js
).
2. Using the wrong useTranslation hook
Problem: In projects that use next‑i18next, you might be tempted to import the useTranslation
hook directly from react‑i18next
. However, it is preferable to import the useTranslation
hook from next‑i18next
instead.
Why?
next‑i18next
wraps react‑i18next
to provide additional server‑side rendering features. In some cases, the react-i18next
version of the hook might not correctly access the initialized i18next
instance. By using the hook from next‑i18next
directly, you can bypass this issue and ensure that your components receive the proper context.
Solution: Simply update your imports:
- import { useTranslation } from 'react-i18next';
+ import { useTranslation } from 'next-i18next';
3. Issues in the Testing Environment
Problem: When running unit tests (for example, with Jest), the i18next
instance may not be initialized before your component renders. This is especially common if you don’t set up the provider in your test environment.
Solution: Either import your i18n initialization file in your test setup (using Jest’s setupFiles
or setupFilesAfterEnv
), or simply mock the translation hook. For example, add this to your Jest setup file:
jest.mock('react-i18next', () => ({
useTranslation: () => ({ t: (key) => key, i18n: {} }),
// you may also want to mock <Trans> if necessary
}));
4. Next.js and Server‑Side Rendering Issues
Problem:
In Next.js projects using next‑i18next
, the error might appear if you use translation hooks inside components that are rendered outside the page-level (for example, in layout components) without proper initialization (via serverSideTranslations
).
Solution:
Ensure that pages which use translations call serverSideTranslations
in their getStaticProps
or getServerSideProps
so that the i18next instance is populated with the correct namespaces. Also make sure you import useTranslation
from next-i18next
rather than directly from react-i18next
to prevent the issue described earlier.
5. Component Library and Multiple Instances
Problem:
If you’re building a component library that uses react-i18next
and then importing that library into a host application, you might end up with multiple copies of i18next
or react-i18next
. This duplication can cause the context to be lost, and the hook won’t see the initialized instance.
Solution:
Use the I18nextProvider to support multiple i18next
instances, like in this example.
In your component library:
import { I18nextProvider, useTranslation } from 'react-i18next';
import i18next from './i18n';
export const i18n = i18next;
function Comp() {
const { t } = useTranslation();
return <div>{t('hello')}</div>;
}
export function MyCompFromOtherLib() {
return (
<I18nextProvider i18n={i18n}>
<Comp />
</I18nextProvider>
);
}
Also a good idea is to propagate the languageChanged
event down to the i18next
instance of the component library:
i18n.on('languageChanged', (lng) => {
i18nOtherLib.changeLanguage(lng);
});
6. Module System (ESM vs. CommonJS) Issues
Problem:
Switching between ESM and CommonJS can sometimes cause subtle issues with module resolution—leading to a situation where your i18next
instance isn’t correctly shared.
Solution:
Double-check that your project’s module settings (e.g. "type": "module"
in package.json) and file extensions align with your bundler’s expectations. In some cases, reverting to CommonJS or ensuring proper aliasing can resolve the issue.
Conclusion
The “You will need to pass in an i18next instance by using initReactI18next” error usually indicates that your translation hook isn’t receiving a properly initialized i18next
instance. Whether it’s due to missing providers, testing setup issues, bundler misconfiguration, or integration challenges in component libraries—the solution always involves ensuring that a single, correctly initialized i18next
instance is available in the React context.
By following the fixes outlined above, you can diagnose your specific scenario and resolve the error — ensuring that your internationalization works smoothly across development, testing, and production environments.
Happy coding — and happy translating! 🚀
Do you want to unleash the full power of i18next? Check out this video.